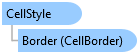
Here is a list of what all can be achieved by using the CellStyle class:
After running this code, the third column of the grid will be painted with a red background. The third row will be painted with a green background. The cell at the intersection will be painted in red, because column styles have priority over row styles. The remaining style elements for these cells (font, alignment, etc) are not defined in the new styles and are automatically inherited from the parent style (CellStyleCollection.Normal).
The cells around the intersection will have a bold font. The style that defines the bold font does not specify a background color, so that element is inherited from the parent style, which may be the "red", "green", or "normal" styles.
private void Form1_Load(object sender, EventArgs e) { _flex = new C1FlexGrid(); _flex.Dock = DockStyle.Fill; this.Controls.Add(_flex); //Bind FlexGrid to DataTable _flex.DataSource = productsTable; CellStyle highReorderLevel = _flex.Styles.Add("HighReorderLevelStyle"); highReorderLevel.BackColor = Color.Green; highReorderLevel.ForeColor = Color.Yellow; CellStyle lowReorderLevel = _flex.Styles.Add("LowReorderLevelStyle"); lowReorderLevel.BackColor = Color.Red; lowReorderLevel.ForeColor = Color.White; _flex.DrawMode = DrawModeEnum.OwnerDraw; _flex.OwnerDrawCell += _flex_OwnerDrawCell; //Applying CellStyle on UnitsInStock Column CellStyle colCellStyle = _flex.Styles.Add("ColumnCellStyle"); NumericUpDown numericUpDownEditor = new NumericUpDown(); numericUpDownEditor.Maximum = 200; colCellStyle.Editor = numericUpDownEditor; _flex.Cols[""UnitsInStock""].Style = colCellStyle; //Customizing the existing Style CellStyle normalStyle = _flex.Styles[CellStyleEnum.Normal]; normalStyle.WordWrap = true; //Applying CellStyle on Rows CellStyle rowCellStyle = _flex.Styles.Add("RowCellStyle"); rowCellStyle.Font= new Font("Calibri", 10, FontStyle.Strikeout); ; for (int row=_flex.Rows.Fixed;row<_flex.Rows.Count;row++) { if (Convert.ToBoolean(_flex[row, "Discontinued"]) == true) _flex.Rows[row].Style = rowCellStyle; } } private void _flex_OwnerDrawCell(object sender, OwnerDrawCellEventArgs e) { //Conditional Formatting using CellStyle if(_flex.Cols[e.Col].Name=="ReorderLevel" && e.Row>0) { if (Convert.ToInt32(_flex[e.Row, e.Col] )> 15) _flex.SetCellStyle(e.Row,e.Col, "HighReorderLevelStyle"); else _flex.SetCellStyle(e.Row, e.Col, "LowReorderLevelStyle"); } }
System.Object
C1.Win.C1FlexGrid.CellStyle