If you wish to use the summary cell to calculate an account balance like a bank passbook (with statements of credits and debits), you need to create your own calculation object which implements the ICalculation interface.
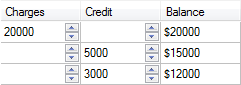
Using Code
The code below uses credit and debit values and returns the balance total in the previous row. If there is no previous row (in case of the first row), it lists the balance as 0.
[VB]
Imports GrapeCity.Win.MultiRow
Public Class CustomCalculation
Implements ICalculation
Private _balanceCellName As String
Private _creditsCellName As String
Private _chargesCellName As String
Public Function Calculate(ByVal context As GrapeCity.Win.MultiRow.CalculationContext) As Object Implements GrapeCity.Win.MultiRow.ICalculation.Calculate
If String.IsNullOrEmpty(Me._creditsCellName) Then Return 0
If String.IsNullOrEmpty(Me._chargesCellName) Then Return 0
If context.Scope = CellScope.Row Then
Dim balance As Decimal = 0
Dim credits As Decimal = 0
Dim charges As Decimal = 0
If Not context.SectionIndex = 0 Then
Dim balanceCellValue As Object = context.GcMultiRow.GetValue(context.SectionIndex - 1, Me._balanceCellName)
balance = DirectCast(balanceCellValue, Decimal)
End If
Dim creditseCellValue As Object = context.GcMultiRow.GetValue(context.SectionIndex, Me._creditsCellName)
If creditseCellValue IsNot Nothing Then
credits = DirectCast(creditseCellValue, Decimal)
End If
Dim chargesCellValue As Object = context.GcMultiRow.GetValue(context.SectionIndex, Me._chargesCellName)
If chargesCellValue IsNot Nothing Then
charges = DirectCast(chargesCellValue, Decimal)
End If
Return balance + charges - credits
End If
Return Nothing
End Function
' Specify cell for Balance
Public Property BalanceCellName() As String
Get
Return _balanceCellName
End Get
Set(ByVal value As String)
_balanceCellName = value
End Set
End Property
' Specify cell for Credit
Public Property CreditsCellName() As String
Get
Return _creditsCellName
End Get
Set(ByVal value As String)
_creditsCellName = value
End Set
End Property
' Specify cell for Charges
Public Property ChargesCellName() As String
Get
Return _chargesCellName
End Get
Set(ByVal value As String)
_chargesCellName = value
End Set
End Property
Public Function Clone() As Object Implements System.ICloneable.Clone
Dim _customCalculation As New CustomCalculation()
_customCalculation.BalanceCellName = Me.BalanceCellName
_customCalculation.CreditsCellName = Me.CreditsCellName
_customCalculation.ChargesCellName = Me.ChargesCellName
Return _customCalculation
End Function
End Class
|
[CS]
using GrapeCity.Win.MultiRow;
public class CustomCalculation : ICalculation
{
private string _balanceCellName;
private string _creditsCellName;
private string _chargesCellName;
public object Calculate(CalculationContext context)
{
if (string.IsNullOrEmpty(this._creditsCellName)) return 0;
if (string.IsNullOrEmpty(this._chargesCellName)) return 0;
if (context.Scope == CellScope.Row)
{
decimal balance = 0;
decimal credits = 0;
decimal charges = 0;
if (context.SectionIndex != 0)
{
object balanceCellValue = context.GcMultiRow.GetValue(context.SectionIndex - 1, this._balanceCellName);
balance = (decimal)balanceCellValue;
}
object creditsCellValue = context.GcMultiRow.GetValue(context.SectionIndex, this._creditsCellName);
if (creditsCellValue != null)
{
credits = (decimal)creditsCellValue;
}
object chargesCellValue = context.GcMultiRow.GetValue(context.SectionIndex, this._chargesCellName);
if (chargesCellValue != null)
{
charges = (decimal)chargesCellValue;
}
return balance + charges - credits;
}
return null;
}
// Specify cell for Balance
public string BalanceCellName
{
get { return _balanceCellName; }
set { _balanceCellName = value; }
}
// Specify cell for Credit
public string CreditsCellName
{
get { return _creditsCellName; }
set { _creditsCellName = value; }
}
// Specify cell for Charges
public string ChargesCellName
{
get { return _chargesCellName; }
set { _chargesCellName = value; }
}
public object Clone()
{
CustomCalculation _customCalculation = new CustomCalculation();
_customCalculation.BalanceCellName = this.BalanceCellName;
_customCalculation.CreditsCellName = this.CreditsCellName;
_customCalculation.ChargesCellName = this.ChargesCellName;
return _customCalculation;
}
}
|
This example creates the template.
[VB]
Imports GrapeCity.Win.MultiRow
Dim charges As New NumericUpDownCell()
charges.Name = "Charges"
Dim credits As New NumericUpDownCell()
credits.Name = "Credit"
Dim balance As New SummaryCell()
balance.Name = "Balance"
Dim custom As New CustomCalculation()
custom.BalanceCellName = "Balance"
custom.ChargesCellName = "Charges"
custom.CreditsCellName = "Credit"
balance.Calculation = custom
balance.Style.TextAlign = MultiRowContentAlignment.MiddleRight
Dim template1 = Template.CreateGridTemplate(New Cell() {charges, credits, balance})
template1.ColumnHeaders(0).Cells(0).Value = "Charges"
template1.ColumnHeaders(0).Cells(1).Value = "Credit"
template1.ColumnHeaders(0).Cells(2).Value = "Balance"
GcMultiRow1.Template = template1
|
[CS]
using GrapeCity.Win.MultiRow;
NumericUpDownCell charges = new NumericUpDownCell();
charges.Name = "Charges";
NumericUpDownCell credits = new NumericUpDownCell();
credits.Name = "Credit";
SummaryCell balance = new SummaryCell();
balance.Name = "Balance";
CustomCalculation custom = new CustomCalculation();
custom.BalanceCellName = "Balance";
custom.ChargesCellName = "Charges";
custom.CreditsCellName = "Credit";
balance.Calculation = custom;
balance.Style.TextAlign = MultiRowContentAlignment.MiddleRight;
Template template1 = Template.CreateGridTemplate(new Cell[] { charges, credits, balance });
template1.ColumnHeaders[0].Cells[0].Value = "Charges";
template1.ColumnHeaders[0].Cells[1].Value = "Credit";
template1.ColumnHeaders[0].Cells[2].Value = "Balance";
gcMultiRow1.Template = template1;
|
See Also