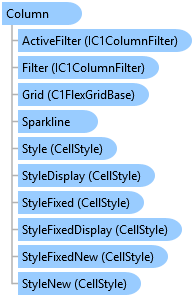
Here is the list of what all can be achieved using the Column class:
private void Form1_Load(object sender, EventArgs e) { _flex = new C1FlexGrid(); _flex.Dock = DockStyle.Fill; this.Controls.Add(_flex); OleDbConnection con = new OleDbConnection("provider=microsoft.jet.oledb.4.0;Data Source="" + Environment.GetFolderPath(Environment.SpecialFolder.Personal) + ""\\ComponentOne Samples\\Common\\C1NWind.mdb"); DataTable productsTable = new DataTable(); DataTable categoryTable = new DataTable(); new OleDbDataAdapter("Select * from Products", con).Fill(productsTable); new OleDbDataAdapter("Select CategoryId,CategoryName,Picture from Categories", con).Fill(categoryTable); _flex.DataSource = productsTable; //Formatting _flex.Cols[""UnitPrice""].Format = "c"; //Applying Custom Editor NumericUpDown numericUpDownEditor = new NumericUpDown(); numericUpDownEditor.Maximum = 200; _flex.Cols["UnitsInStock"].Editor = numericUpDownEditor; //Using ImageMap ListDictionary categoryList = new ListDictionary(); foreach (DataRow row in categoryTable.Rows) categoryList.Add(row["CategoryId"] ,row["CategoryName"]); ImageList imgList = new ImageList(); foreach (DataRow row in categoryTable.Rows) imgList.Images.Add(row[""CategoryId""].ToString(), LoadImage((byte[])(row["Picture"]))); ListDictionary images = new ListDictionary(); for (int i = 1; i <=imgList.Images.Count; i++) images[i] = imgList.Images[i.ToString()]; _flex.Cols["CategoryId"].DataMap = categoryList; _flex.Cols["CategoryId"].ImageMap = images; _flex.Cols["CategoryId"].ImageAndText = true; _flex.Cols["CategoryId"].ImageAlign = ImageAlignEnum.RightBottom; //Applying filter _flex.AllowFiltering = true; ConditionFilter priceFilter = new ConditionFilter(); priceFilter.Condition1.Parameter = "50"; priceFilter.Condition1.Operator = ConditionOperator.GreaterThan; _flex.Cols["UnitPrice"].Filter = priceFilter; //Column calculation on FlexGrid columns through expressions. Column unboundcol = _flex.Cols.Add(); unboundcol.DataType = typeof(object); unboundcol.AllowEditing = false; unboundcol.Name = "CustomColumn"; unboundcol.Caption = "Custom Column"; unboundcol.AllowExpressionEditing = true; unboundcol.Expression = "[UnitsInStock] -[UnitsOnOrder]"; //Sorting a specific column _flex.Cols["ReorderLevel"].Sort = SortFlags.Descending; _flex.Sort(SortFlags.UseColSort, _flex.Cols["ReorderLevel"].Index ); } static Image LoadImage(byte[] picData) { // make sure this is an embedded object const int bmData = 78; if (picData == null || picData.Length < bmData + 2) return null; if (picData[0] != 0x15 || picData[1] != 0x1c) return null; // we only handle bitmaps for now if (picData[bmData] != 'B' || picData[bmData + 1] != 'M') return null; // load the picture Image img = null; try { MemoryStream ms = new MemoryStream(picData, bmData, picData.Length - bmData); img = Image.FromStream(ms); } catch { } // return what we got return img; }
System.Object
C1.Win.C1FlexGrid.RowCol
C1.Win.C1FlexGrid.Column